|
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253545556575859606162636465666768697071727374757677787980 |
- ## Notes
- - The app is deployed to test.onyeka.ca
- - I updated react and other packages because 16.7 was being used, and hooks are
- now the simplified method of managing state in react.
-
- ### TODO
- - Show dates on calls?
- - Add date seperators to calls
- - Sort calls by descending dates
- - Add missed and voicemail icons to represent status
- - Give call entries shine and box shadow. Maybe color them by type
- - Add entry transition animation
- - Add favicon
- - Seperator could be added by putting li renderer in a seperate function from
- Feed
-
- ## Summary
-
- The goal of this test is to make you code a small ReactJS app. We have prepared a skeleton app for you, but please change whatever you want (CSS files, HTML structure, JS structure, etc).
-
- The app will have two different components:
- - **Activity Feed** - simple list of calls
- - **Activity Detail** - detail of a call
- - **Archive** - the final user should be able to archive (and unarchive) a call. Archived calls will no longer be displayed on the Activity Feed and should have a separate Archived Tab.
-
- Show us what you can do in 24 hours. You will be assessed on the following parameters:
- - Focus on design (Pay attention to the UI/UX and transitions)
- - Best React Practices
- - Code Readability and Maintainability
-
- ## Submission
- After you're done with the assignment, please submit a link to the **GitHub/Bitbucket repository** (make sure it's public) with your code **AND** a deployment link where our recruiters can interact with the live version. You can use freely available tools like **Netlify, Vercel, Heroku, etc** to deploy your React application.
-
- You should email both the links to our recruiters with the subject line **Full Stack Developer Assessment - [Your Name]**
-
- **Note:** Submissions without a valid repository and deployment link will be removed from any further consideration.
-
- To give you an idea, here's what our app looks like:
-
-
- 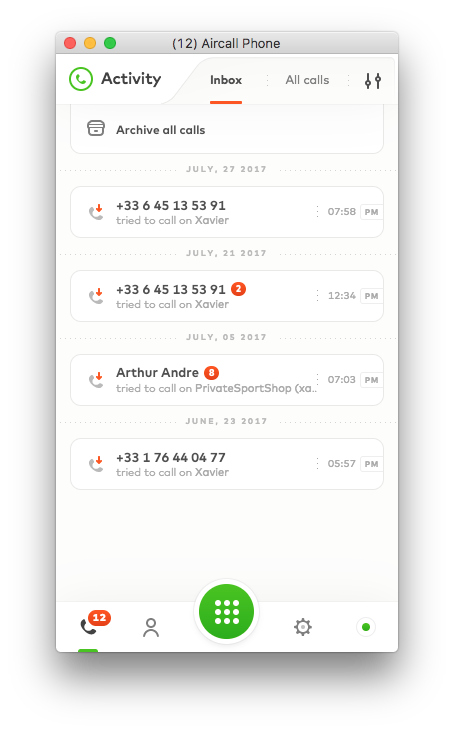
-
- ## Installation
-
- We're using [yarn](https://yarnpkg.com) here (but you can use npm):
-
- ```
- yarn install
- yarn start
- ```
-
- ## API documentation
-
- ### Routes
-
- Here is the API address: https://aircall-job.herokuapp.com.
-
- As you can see, it's hosted on a free Heroku server, which means that the first time you will fetch the API, it will take few seconds to answer.
-
- - **GET** - https://aircall-job.herokuapp.com/activities: get calls to display in the Activity Feed
- - **GET** - https://aircall-job.herokuapp.com/activities/:id: retrieve a specific call details
- - **POST** - https://aircall-job.herokuapp.com/activities/:id: update a call. The only field updatable is `is_archived (bool)`. You'll need to send a JSON in the request body:
- ```
- {
- is_archived: true
- }
- ```
- - **GET** - https://aircall-job.herokuapp.com/reset: Reset all calls to initial state (usefull if you archived all calls).
-
- ### Call object
-
- - **id** - unique ID of call
- - **created_at** - creation date
- - **direction** - `inbound` or `outbound` call
- - **from** - caller's number
- - **to** - callee's number
- - **via** - Aircall number used for the call
- - **duration** - duration of a call (in seconds)
- - **is_archived** - call is archived or not
- - **call_type** - can be a `missed`, `answered` or `voicemail` call.
|